a weekend love story - raylib/zig
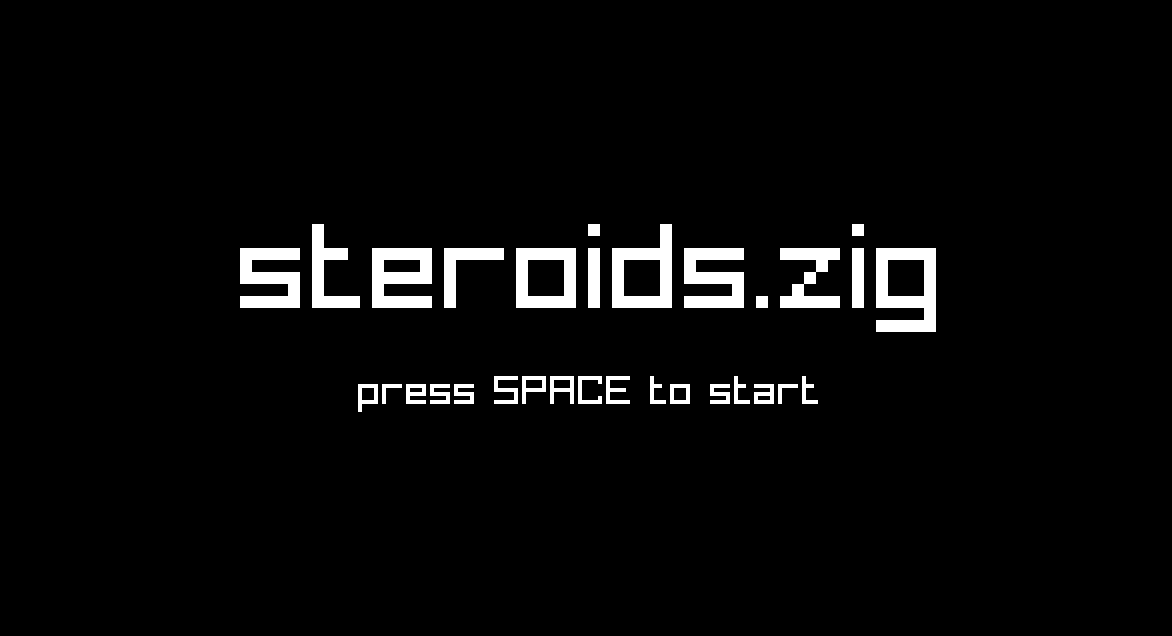
Zig Psyop
Before this weekend, I was a plebeian who used JavaScript for 80% of my tasks. Now I am an esoteric plebeian who has used zig once. Anyway, I decided to give zig a shot and try to build a game with it. There was really only two libraries I wanted to learn (sokol and raylib), I went with raylib.
Initial References
I went through the official raylib docs to get a feel for the API, then I searched for zig bindings and found two, Not-Nik/raylib-zig and ryupold/raylib-zig
The second one hasn’t been updated in 6 months, and it isn’t using the zig package manager. Ergo, leaving me no choice but to use the first one.
Setup
Fairly easy to set things up, I just needed to install zig and raylib-zig.
NOTE : We will be using zig v0.12.0 instead of v0.13.0, we will see why later.
I used zvm to make life easier mangaging various zig versions, but you can install zig however you want.
Follow this part of the docs to install raylib-zig. Do this inside your game directory. This adds raylib-zig as a dependency to our project. You can check the build.zig.zon
file to verify.
Now the most important part if you want to compile your game for the web with emscripten. IF YOU’RE NOT BUILDING FOR THE WEB, PLEASE SKIP.
Install emsdk
as mentioned here: emscripten installation guide and make sure to do the source ./emsdk_env.sh
command.
NOTE : Just make sure not to clone the emsdk into $HOME/.emscripten, because emscripten uses that as the default cache directory. It will fuck your build up. (I didn’t do this at all)
If you followed the raylib-zig installation guide, your build.zig should look something like this:
const std = @import("std");
pub fn build(b: *std.Build) !void {
const target = b.standardTargetOptions(.{});
const optimize = b.standardOptimizeOption();
const exe = b.addExecutable(.{
.name = "steroids.zig",
.root_source_file = b.path("src/main.zig"),
.target = target,
.optimize = optimize,
});
const raylib_dep = b.dependency("raylib-zig", .{
.target = target,
.optimize = optimize,
});
const raylib = raylib_dep.module("raylib");
const raylib_artifact = raylib_dep.artifact("raylib");
.linkLibrary(raylib_artifact);
exe.root_module.addImport("raylib", raylib);
exe
.installArtifact(exe);
b
const run_cmd = b.addRunArtifact(exe);
.step.dependOn(b.getInstallStep());
run_cmd
if (b.args) |args| {
.addArgs(args);
run_cmd
}
const run_step = b.step("run", "Run the app");
.dependOn(&run_cmd.step);
run_step }
If it isn’t, it should be now! Now we need to add a block specific to the emscripten target.
// add these two imports
const fs = std.fs;
const rlz = @import("raylib-zig");
pub fn build(b: *std.Build) !void {
// ... other code
const raylib = raylib_dep.module("raylib");
const raylib_artifact = raylib_dep.artifact("raylib");
if (target.query.os_tag == .emscripten) {
const exe_lib = rlz.emcc.compileForEmscripten(b, "steroids.zig", "src/main.zig", target, optimize);
.linkLibrary(raylib_artifact);
exe_lib.root_module.addImport("raylib", raylib);
exe_lib
const include_path = try fs.path.join(b.allocator, &.{ b.sysroot.?, "cache", "sysroot", "include" });
defer b.allocator.free(include_path);
.addIncludePath(.{ .path = include_path });
exe_lib.linkLibC();
exe_lib
// linking raylib to the exe_lib output file.
const link_step = try rlz.emcc.linkWithEmscripten(b, &[_]*std.Build.Step.Compile{ exe_lib, raylib_artifact });
// Use the custom HTML template
// This will be the index.html where the game is rendered.
// You can find an example in my repository.
.addArg("--shell-file");
link_step.addArg("shell.html");
link_step
// Embed the assets directory
// This generates an index.data file which is neede for the game to run.
.addArg("--preload-file");
link_step.addArg("assets");
link_step
.addArg("-sALLOW_MEMORY_GROWTH");
link_step.addArg("-sWASM_MEM_MAX=16MB");
link_step.addArg("-sTOTAL_MEMORY=16MB");
link_step.addArg("-sERROR_ON_UNDEFINED_SYMBOLS=0");
link_step// Add any other flags you need
.getInstallStep().dependOn(&link_step.step);
bconst run_step = try rlz.emcc.emscriptenRunStep(b);
.step.dependOn(&link_step.step);
run_stepconst run_option = b.step("run", "Run the game");
.dependOn(&run_step.step);
run_optionreturn;
}
// rest of the build script
}
I missed this code block and was stuck trying to figure out how to get the emscripten run step to work. :(
Write some code!
I wrote an asteroids clone following along with this video: zig space rocks - jdh. Go write whatever game you want!
Here is a screenshot of the game in action:
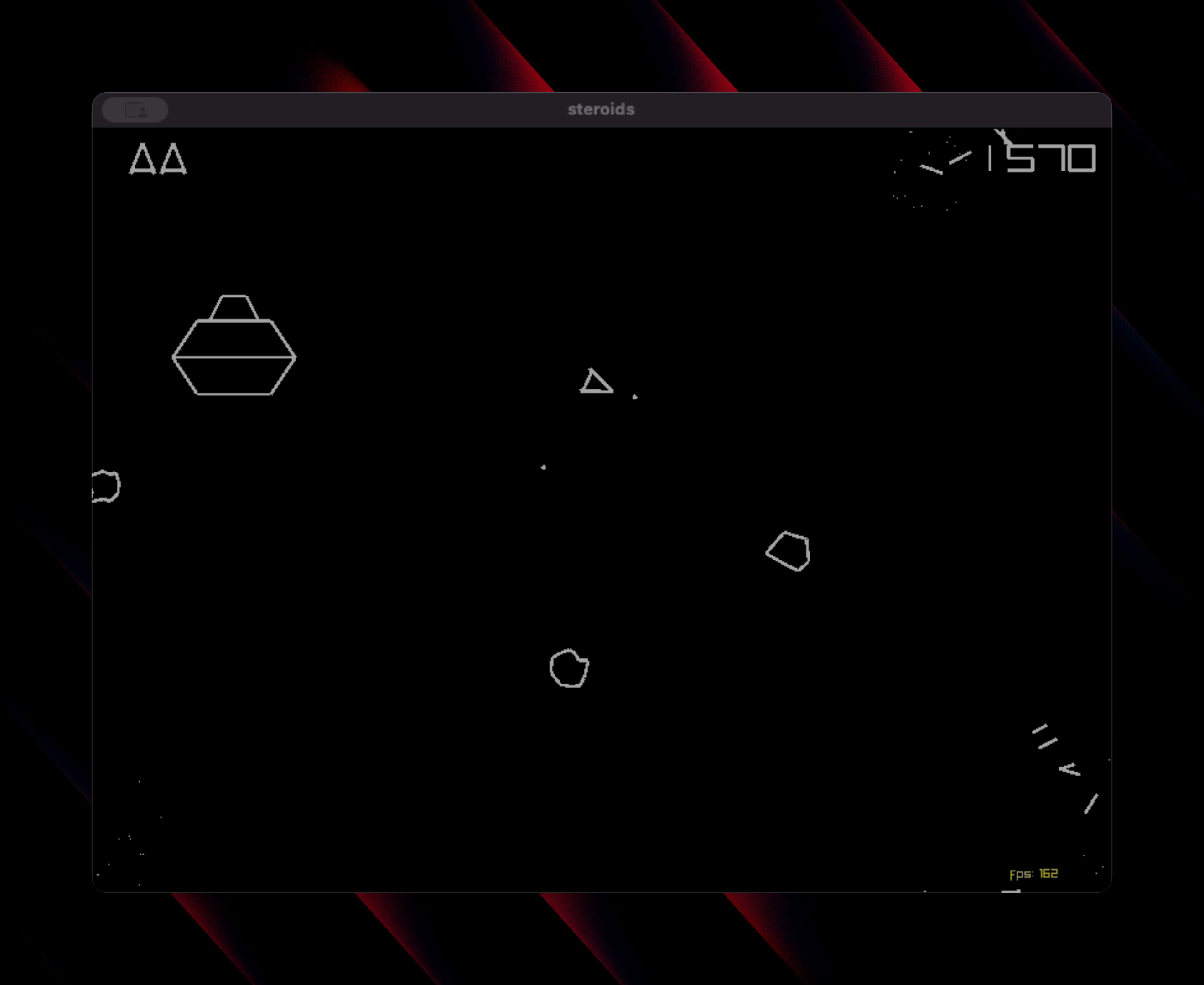
Here are some other cool games made in zig: tetris, terrain-zigger
Below is some cool zig code I wrote that I quite like:
.initAudioDevice();
rldefer rl.closeAudioDevice();
= Sound{
sound .asteroid = rl.loadSound("assets/asteroid.wav"),
.bloop_lo = rl.loadSound("assets/bloop_lo.wav"),
.bloop_hi = rl.loadSound("assets/bloop_hi.wav"),
.explode = rl.loadSound("assets/explode.wav"),
.shoot = rl.loadSound("assets/shoot.wav"),
.thrust = rl.loadSound("assets/thrust.wav"),
};
.setSoundVolume(sound.explode, 0.5);
rl
// this unwraps the loop at compile time for each field in the Sound struct
// and frees the memory allocated for each sound
defer inline for (std.meta.fields(Sound)) |f| {
.unloadSound(@field(sound, f.name));
rl
};
defer state.asteroids.deinit();
defer state.asteroids_q.deinit();
defer state.particles.deinit();
defer state.projectiles.deinit();
defer state.aliens.deinit();
This defer
syntax is so fucking cool, I will never forget to free
again.
const bloop_intensity: usize = @min(@as(usize, @intFromFloat(state.now - state.stage_start)) / 16, 4);
const bloop_mod: usize = 60;
const adjusted_bloop_mod: usize = @max(1, bloop_mod >> @intCast(bloop_intensity));
if (state.frame % adjusted_bloop_mod == 0) {
.bloop += 1;
state
}
if (!state.ship.isDead() and state.bloop != state.last_bloop) {
.playSound(if (state.bloop % 2 == 0) sound.bloop_hi else sound.bloop_lo);
rl
}.last_bloop = state.bloop; state
This code is for deciding when to play the low/high bloop sound. It increases in intensity the longer a player is alive. Makes it feel like the game is getting harder. Pretty cool! Also avoided for loops with bit shifting. absolutely unnecessary. But I did it anyway.
Memory management
One thing I think is important is for some reason I wasn’t able to just use a std.heap.GeneralPurposeAllocator
for the heap allocations. So i reused some code from the ryupold/examples-raylib.zig repo and made a custom emscripten allocator.
// i modified only one line
// line 50
const c = @cImport({
@cDefine("__EMSCRIPTEN__", "1");
@cInclude("emscripten.h"); // <- I changed it to just emscripten.h
@cInclude("stdlib.h");
});
Without this, I was getting Out of Memory
issues. I also increased the memory limit to 16MB. Idk which of those fixed it, but it works now! After that, I was sitting at a solid 1.23 MB
of memory usage, so I think I was good.
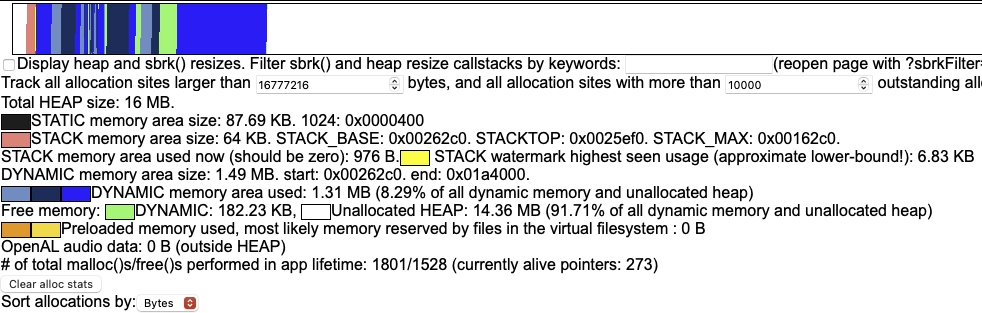
Build the game
You can build the game for desktop with zig build run
. For the web, you need to run the following command:
zig build -Dtarget=wasm32-emscripten --sysroot "/absolute/path/to/emsdk/upstream/emscripten"
# the absolute path is crucial, otherwise it will not work.
# now to run the game, you can use the following command:
emrun ./zig-out/htmlout/index.html
Now, as to why we used v0.12.0
. For some reason the build command above fails for the emscripten target with v0.13.0
as mentioned in this open issue. If someone can figure out why, please mention it in the issue!
You can drop everything in the zig-out/htmlout
folder and host it wherever.
Fin!
Was a fun start to my zig arc! I hope you guys don’t waste time debugging shit like me lol.
May we zig harder every day.